http://www.warriorpoint.com/blog/2009/05/26/android-switching-screens-in-an-activity-with-animations-using-viewflipper/
http://www.careerride.com/android-interview-questions.aspx
http://www.helloandroid.com/content/useful-java-apis-android-applications
http://www.slideshare.net/peterbuck/an-introduction-to-android-4004533?src=related_normal&rel=858298
http://rayfd.wordpress.com/2007/05/20/10-eclipse-navigation-shortcuts-every-java-programmer-should-know/
http://kevindion.com/2011/01/custom-xml-attributes-for-android-widgets/
http://stackoverflow.com/questions/2376250/custom-fonts-and-xml-layouts-android
http://stackoverflow.com/questions/4732634/how-to-set-the-header-and-footer-for-linear-layout-in-android
http://coderzheaven.com/2011/04/expandable-listview-in-android-using-simpleexpandablelistadapter-a-simple-example/
http://arstechnica.com/civis/viewtopic.php?f=20&t=1108192&view=unread
http://www.portalandroid.org/comunidade/search.php?search_id=active_topics
http://thinkandroid.wordpress.com/
http://mylifewithandroid.blogspot.com/search?q=map&updated-max=2008-02-20T22%3A11%3A00%2B01%3A00&max-results=20
http://illuminatus.oczombies.net/?p=78
http://marakana.com/forums/android/examples/
Thursday, September 29, 2011
Wednesday, September 21, 2011
Query and parse Google+ JSON using Google+ API.
There is a post in The official Google Code blog, discuss about Getting started on the Google+ API. It's a exercise to query and parse Google+ JSON using Google+ API.
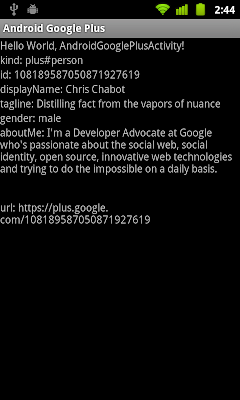
To use Google+ API, you have to
note: have to modify AndroidManifest.xml to grant permission of "android.permission.INTERNET".
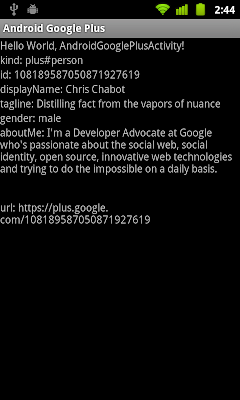
To use Google+ API, you have to
package com.exercise.AndroidGooglePlus;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.Bundle;
import android.text.Html;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidGooglePlusActivity extends Activity {
TextView item_kind;
TextView item_id;
TextView item_displayName;
TextView item_tagline;
TextView item_gender;
TextView item_aboutMe;
TextView item_url;
final static String GooglePlus_url
= "https://www.googleapis.com/plus/v1/people/108189587050871927619?key=<your own Google+ API key>";
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
item_kind = (TextView)findViewById(R.id.item_kind);
item_id = (TextView)findViewById(R.id.item_id);
item_displayName = (TextView)findViewById(R.id.item_displayName);
item_tagline = (TextView)findViewById(R.id.item_tagline);
item_gender = (TextView)findViewById(R.id.item_gender);
item_aboutMe = (TextView)findViewById(R.id.item_aboutMe);
item_url = (TextView)findViewById(R.id.item_url);
String googlePlusResult = QueryGooglePlus();
ParseGooglePlusJSON(googlePlusResult);
}
String QueryGooglePlus(){
String qResult = null;
HttpClient httpClient = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(GooglePlus_url);
try {
HttpEntity httpEntity = httpClient.execute(httpGet).getEntity();
if (httpEntity != null){
InputStream inputStream = httpEntity.getContent();
Reader in = new InputStreamReader(inputStream);
BufferedReader bufferedreader = new BufferedReader(in);
StringBuilder stringBuilder = new StringBuilder();
String stringReadLine = null;
while ((stringReadLine = bufferedreader.readLine()) != null) {
stringBuilder.append(stringReadLine + "\n");
}
qResult = stringBuilder.toString();
}
} catch (ClientProtocolException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
} catch (IOException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
}
return qResult;
}
void ParseGooglePlusJSON(String json){
if(json != null){
try {
JSONObject JsonObject = new JSONObject(json);
item_kind.setText("kind: " + JsonObject.getString("kind"));
item_id.setText("id: " + JsonObject.getString("id"));
item_displayName.setText("displayName: " + JsonObject.getString("displayName"));
item_tagline.setText("tagline: " + JsonObject.getString("tagline"));
item_gender.setText("gender: " + JsonObject.getString("gender"));
item_aboutMe.setText("aboutMe: " + Html.fromHtml(JsonObject.getString("aboutMe")));
item_url.setText("url: " + JsonObject.getString("url"));
Toast.makeText(AndroidGooglePlusActivity.this,
"finished",
Toast.LENGTH_LONG).show();
} catch (JSONException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
}
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:id="@+id/item_kind"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_id"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_displayName"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_tagline"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_gender"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_aboutMe"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_url"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
note: have to modify AndroidManifest.xml to grant permission of "android.permission.INTERNET".
Apply your own Google+ API key
Apply your own Google+ API key
The official Google Code blog have a new post about Getting started on the Google+ API. Google+ gives users full control over their information, supporting everything from intimate conversations with family to public showcases and debates. This initial API release is focused on public data only — it lets you read information that people have shared publicly on Google+.In order to read information from Google+, you have to apply your API Key. Visit APIs Console, you have to login your Google account to create your API key.
- Click on the API project on the left and select Create...
- Enter the name of your project and click Create project.
- Click to enable Google+ API, you will be asked to review and accept the terms of service.
- Click API Access, your API Key will be found.
Now you can copy the link with your own API key at browser to read the JSON returned from Google+.
https://www.googleapis.com/plus/v1/people/108189587050871927619?key=<your API key>
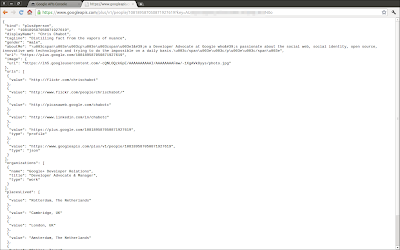
XML PARSING SAMPLE IN ANDROID
XML PARSING SAMPLE IN ANDROID
In this exercise, we read our own XML Resource (in /res/xml folder) using XmlResourceParser, and display the contents on screen.
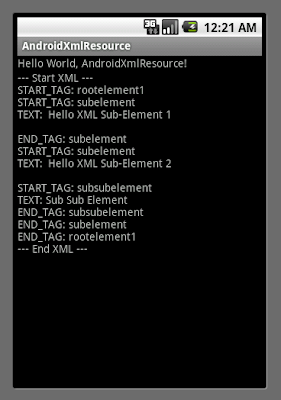
First of all, create a folder /res/xml, and our own XML file myxml.xml
Modify main.xml
Finally, modify java code
In this exercise, we read our own XML Resource (in /res/xml folder) using XmlResourceParser, and display the contents on screen.
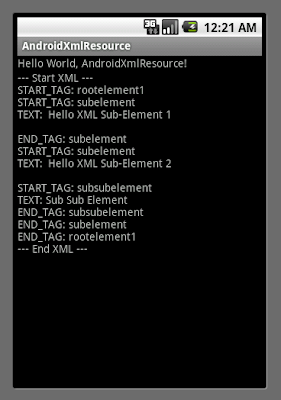
First of all, create a folder /res/xml, and our own XML file myxml.xml
<?xml version="1.0" encoding="utf-8"?>
<rootelement1>
<subelement>
Hello XML Sub-Element 1
</subelement>
<subelement>
Hello XML Sub-Element 2
<subsubelement>Sub Sub Element</subsubelement>
</subelement>
</rootelement1>
Modify main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/my_xml"
/>
</LinearLayout>
Finally, modify java code
package com.exercise.AndroidXmlResource;
import java.io.IOException;
import org.xmlpull.v1.XmlPullParser;
import org.xmlpull.v1.XmlPullParserException;
import android.app.Activity;
import android.content.res.Resources;
import android.content.res.XmlResourceParser;
import android.os.Bundle;
import android.widget.TextView;
public class AndroidXmlResource extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView myXmlContent = (TextView)findViewById(R.id.my_xml);
String stringXmlContent;
try {
stringXmlContent = getEventsFromAnXML(this);
myXmlContent.setText(stringXmlContent);
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private String getEventsFromAnXML(Activity activity)
throws XmlPullParserException, IOException
{
StringBuffer stringBuffer = new StringBuffer();
Resources res = activity.getResources();
XmlResourceParser xpp = res.getXml(R.xml.myxml);
xpp.next();
int eventType = xpp.getEventType();
while (eventType != XmlPullParser.END_DOCUMENT)
{
if(eventType == XmlPullParser.START_DOCUMENT)
{
stringBuffer.append("--- Start XML ---");
}
else if(eventType == XmlPullParser.START_TAG)
{
stringBuffer.append("\nSTART_TAG: "+xpp.getName());
}
else if(eventType == XmlPullParser.END_TAG)
{
stringBuffer.append("\nEND_TAG: "+xpp.getName());
}
else if(eventType == XmlPullParser.TEXT)
{
stringBuffer.append("\nTEXT: "+xpp.getText());
}
eventType = xpp.next();
}
stringBuffer.append("\n--- End XML ---");
return stringBuffer.toString();
}
}
A simple RSS reader, using Android's org.xml.sax package.
A simple RSS reader, using Android's org.xml.sax package.
In order to make it simple, only the contents under "title" tag will be retrieved and appended as a string.

To allow the Android application, "android.permission.INTERNET" have to be granted to the application. Modify AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.exercise.AndroidRssReader"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".AndroidRssReader"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
<uses-sdk android:minSdkVersion="4" />
<uses-permission android:name="android.permission.INTERNET" />
</manifest>
Modify main.xml to add a TextView to display the result.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<ScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/result" />
</ScrollView>
</LinearLayout>
Java source code.
package com.exercise.AndroidRssReader;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.Attributes;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.DefaultHandler;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
public class AndroidRssReader extends Activity {
String streamTitle = "";
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView result = (TextView)findViewById(R.id.result);
try {
URL rssUrl = new URL("http://example.com/example.xml.........");
SAXParserFactory mySAXParserFactory = SAXParserFactory.newInstance();
SAXParser mySAXParser = mySAXParserFactory.newSAXParser();
XMLReader myXMLReader = mySAXParser.getXMLReader();
RSSHandler myRSSHandler = new RSSHandler();
myXMLReader.setContentHandler(myRSSHandler);
InputSource myInputSource = new InputSource(rssUrl.openStream());
myXMLReader.parse(myInputSource);
result.setText(streamTitle);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
result.setText("Cannot connect RSS!");
} catch (ParserConfigurationException e) {
// TODO Auto-generated catch block
e.printStackTrace();
result.setText("Cannot connect RSS!");
} catch (SAXException e) {
// TODO Auto-generated catch block
e.printStackTrace();
result.setText("Cannot connect RSS!");
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
result.setText("Cannot connect RSS!");
}
}
private class RSSHandler extends DefaultHandler
{
final int stateUnknown = 0;
final int stateTitle = 1;
int state = stateUnknown;
int numberOfTitle = 0;
String strTitle = "";
String strElement = "";
@Override
public void startDocument() throws SAXException {
// TODO Auto-generated method stub
strTitle = "--- Start Document ---\n";
}
@Override
public void endDocument() throws SAXException {
// TODO Auto-generated method stub
strTitle += "--- End Document ---";
streamTitle = "Number Of Title: " + String.valueOf(numberOfTitle) + "\n"
+ strTitle;
}
@Override
public void startElement(String uri, String localName, String qName,
Attributes attributes) throws SAXException {
// TODO Auto-generated method stub
if (localName.equalsIgnoreCase("title"))
{
state = stateTitle;
strElement = "Title: ";
numberOfTitle++;
}
else
{
state = stateUnknown;
}
}
@Override
public void endElement(String uri, String localName, String qName)
throws SAXException {
// TODO Auto-generated method stub
if (localName.equalsIgnoreCase("title"))
{
strTitle += strElement + "\n";
}
state = stateUnknown;
}
@Override
public void characters(char[] ch, int start, int length)
throws SAXException {
// TODO Auto-generated method stub
String strCharacters = new String(ch, start, length);
if (state == stateTitle)
{
strElement += strCharacters;
}
}
}
}
Tuesday, September 20, 2011
Lifecycle of an Android Application
Lifecycle of an Android Application
In most cases, every Android application runs in its own Linux process. This process is created for the application when some of its code needs to be run, and will remain running until it is no longer needed and the system needs to reclaim its memory for use by other applications.
An important and unusual feature of Android is that an application process's lifetime is not directly controlled by the application itself. Instead, it is determined by the system through a combination of the parts of the application that the system knows are running, how important these things are to the user, and how much overall memory is available in the system.
It is important that application developers understand how different application components (in particular Activity, Service, and IntentReceiver) impact the lifetime of the application's process. Not using these components correctly can result in the system killing the application's process while it is doing important work.
A common example of a process lifecycle bug is an IntentReceiver that starts a thread when it receives an Intent in its onReceiveIntent() method, and then returns from the function. Once it returns, the system considers that IntentReceiver to be no longer active, and thus its hosting process no longer needed (unless other application components are active in it). Thus, it may kill the process at any time to reclaim memory, terminating the spawned thread that is running in it. The solution to this problem is to start a Service from the IntentReceiver, so the system knows that there is still active work being done in the process.
To determine which processes should be killed when low on memory, Android places them into an "importance hierarchy" based on the components running in them and the state of those components. These are, in order of importance:
1. A foreground process is one holding an Activity at the top of the screen that the user is interacting with (its onResume() method has been called) or an IntentReceiver that is currently running (its onReceiveIntent() method is executing). There will only ever be a few such processes in the system, and these will only be killed as a last resort if memory is so low that not even these processes can continue to run. Generally at this point the device has reached a memory paging state, so this action is required in order to keep the user interface responsive.
2. A visible process is one holding an Activity that is visible to the user on-screen but not in the foreground (its onPause() method has been called). This may occur, for example, if the foreground activity has been displayed with a dialog appearance that allows the previous activity to be seen behind it. Such a process is considered extremely important and will not be killed unless doing so is required to keep all foreground processes running.
3. A service process is one holding a Service that has been started with the startService() method. Though these processes are not directly visible to the user, they are generally doing things that the user cares about (such as background mp3 playback or background network data upload or download), so the system will always keep such processes running unless there is not enough memory to retain all foreground and visible process.
4. A background process is one holding an Activity that is not currently visible to the user (its onStop() method has been called). These processes have no direct impact on the user experience. Provided they implement their activity lifecycle correctly (see Activity for more details), the system can kill such processes at any time to reclaim memory for one of the three previous processes types. Usually there are many of these processes running, so they are kept in an LRU list to ensure the process that was most recently seen by the user is the last to be killed when running low on memory.
5.An empty process is one that doesn't hold any active application components. The only reason to keep such a process around is as a cache to improve startup time the next time a component of its application needs to run. As such, the system will often kill these processes in order to balance overall system resources between these empty cached processes and the underlying kernel caches.
When deciding how to classify a process, the system picks the most important level of all the components currently active in the process. See the Activity, Service, and IntentReceiver documentation for more detail on how each of these components contribute to the overall lifecycle of a process. The documentation for each of these classes describes in more detail how they impact the overall lifecycle of their application.
Sample Android Activity,Service ( 1 )
An activity is the equivalent of a Frame/Window in GUI toolkits. It takes up the entire drawable area of the screen. An activity is what gets bound to the AndroidManifest.xml as the main entry point into an application. For long running tasks, it’s best to use a service that talks to this activity.
Service : Activities are meant to display the UI and get input from the user. Services on the other hand keep running for the duration of the user’s ‘session’ on the device.
Activity Navigation:
This has done by the following two ways.
1. Fire and forget – create an event (Intent) and fire it
2. Async callback – create an event (Intent), fire it, and wait for it’s response in a callback method (of the calling-Activity).
Activity history stack
Please note that Android maintains a history stack of all the Activities that have been spawned in an application’s Linux process. In the sample code above, the calling-Activity simply provides the class name of the sub-Activity. When the sub-Activity finishes, it puts it’s result code and any data back on the stack and finishes itself. Since Android maintains the stack, it knows which calling Activity to pass the result back to. The calling-Activity has an onActivityResult method that handles all the callbacks from the sub-Activities. This is pretty confusing at first, since to keep it all straight we have to use CorrelationIds (more on that below).
Example : User wants to navigate Talk application to Browser Application. Using the Browser application wants to serach some photos and saved his local memory. After that he shares the photo with his friend via email application and finally he will come back to the talk application.
Firstly, as the user is talking to his friend, a specific Talk application is opened, which contains the activity manager. System Creates two processes, the main system process and Talk application process. Moreover, before going to Web Browser application, the system saves a Talk state T in order to remember that process. At this point, as a user holds a talk and opens a web browser, the system creates a new process and new web browser activity is launched in it. Again, the state of the last activity is saved.After that, the user browses the internet, finds his picture in Picasa album and saves it to particular folder. He does not close a web browser, instead he opens a folder to find saved picture. The folder activity is launched in particular process. At this point, the user finds his saved picture in the folder and he creates a request to open an Email application. The last state F is saved. Now assume that the mobile phone is out of the memory and there is no room to create a new process for Email application. Therefore, Android looks to kill a process. It can not destroy Folder process, as it was used previously and could be reused again, so it kills Web Browser process as it is not useful anymore and locates a new Email process instead. The user opens Email application and sends a picture to his friend via email. Now he wants to go back to the Talk application and to resume a talk to his friend. Because of the previously saved states, this work is done fast and easily. In this example, Email application is popped out and the user sees a previous Folder application.Next, the user goes back to Web Browser application. Unfortunately, web browser
process was killed previously so the system has to kill another process (in our case it is Email application process, which is not used anymore) in order to locate Web Browser process and manage the stack memory. Now the user comes back to the Talk application and resumes his talk with his friend. Because of the saved states, going back procedure is fast and useful, because it remembers previous activities and its views.
This example shows, that it does not matter how many applications and processes are active or how much available memory is left, Android it manages fast and without a user interaction.
Fire and forget
For this method we just calls the child activity and flow the process. we are not getting any return value from the child activity. Here intent is just an event. It can have a target of an activity calss along with some date that's passed in via a Bundle.
refer : http://about-android.blogspot.com/2009/12/passing-data-or-parameter-to-another_02.html
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
3. Call the child Activity from mainactivity with the bundle
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
4. Get the Value from MainActivity in ChildActivity
this.getIntent().getExtras().getString("sample")
5. Toast the Message
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
}
}
Async callback, and correlationId
The calling-Activity has to provide a correlationId/request code to the Intent/event, before firing/raising it. This is then used by the sub-Activity to report it’s results back to the calling-Activity when it’s ready. The calling-Activity does not stop when it spawns the sub-Activity.
Please note that this sub-Activity is not "modal", that is, the calling Activity does not block, when startSubActivity() is called. So if you’re thinking that this is like a modal dialog box, where the calling-Activity will wait for the sub-Activity to produce a result, then you have to think of it differently.
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Call the child Activity from mainactivity with the bundle
Intent i = new Intent(this, ChildActivity.class);
startActivityForResult(i, 1);
4. Set the Value from ChildActivity To MainActivity
setResult(RESULT_OK, new Intent().putExtra("result", "value from Child Activity"));
finish();
5. Receive the value from ChildActiivity and Print the Message in onActivityResult Method
if (resultCode == RESULT_OK) {
String name = data.getStringExtra("result");
Toast.makeText(this, "You have chosen the City: " + " " + name,
Toast.LENGTH_LONG).show();
}
Source Code
MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.Toast;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Intent i = new Intent(this, ChildActivity.class);
startActivityForResult(i, 1);
}
protected void start(Intent intent) {
this.startActivityForResult(intent, 1);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (resultCode == RESULT_OK) {
String name = data.getStringExtra("result");
Toast.makeText(this, "You have chosen the Value: " + " " + name,
Toast.LENGTH_LONG).show();
}
super.onActivityResult(requestCode, resultCode, data);
}
}
ChildActivity.java
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, "Child Activity", Toast.LENGTH_LONG).show();
setResult(RESULT_OK, new Intent().putExtra("result", "value from Child Activity"));
finish();
}
}
Service : Activities are meant to display the UI and get input from the user. Services on the other hand keep running for the duration of the user’s ‘session’ on the device.
Activity Navigation:
This has done by the following two ways.
1. Fire and forget – create an event (Intent) and fire it
2. Async callback – create an event (Intent), fire it, and wait for it’s response in a callback method (of the calling-Activity).
Activity history stack
Please note that Android maintains a history stack of all the Activities that have been spawned in an application’s Linux process. In the sample code above, the calling-Activity simply provides the class name of the sub-Activity. When the sub-Activity finishes, it puts it’s result code and any data back on the stack and finishes itself. Since Android maintains the stack, it knows which calling Activity to pass the result back to. The calling-Activity has an onActivityResult method that handles all the callbacks from the sub-Activities. This is pretty confusing at first, since to keep it all straight we have to use CorrelationIds (more on that below).
Example : User wants to navigate Talk application to Browser Application. Using the Browser application wants to serach some photos and saved his local memory. After that he shares the photo with his friend via email application and finally he will come back to the talk application.
Firstly, as the user is talking to his friend, a specific Talk application is opened, which contains the activity manager. System Creates two processes, the main system process and Talk application process. Moreover, before going to Web Browser application, the system saves a Talk state T in order to remember that process. At this point, as a user holds a talk and opens a web browser, the system creates a new process and new web browser activity is launched in it. Again, the state of the last activity is saved.After that, the user browses the internet, finds his picture in Picasa album and saves it to particular folder. He does not close a web browser, instead he opens a folder to find saved picture. The folder activity is launched in particular process. At this point, the user finds his saved picture in the folder and he creates a request to open an Email application. The last state F is saved. Now assume that the mobile phone is out of the memory and there is no room to create a new process for Email application. Therefore, Android looks to kill a process. It can not destroy Folder process, as it was used previously and could be reused again, so it kills Web Browser process as it is not useful anymore and locates a new Email process instead. The user opens Email application and sends a picture to his friend via email. Now he wants to go back to the Talk application and to resume a talk to his friend. Because of the previously saved states, this work is done fast and easily. In this example, Email application is popped out and the user sees a previous Folder application.Next, the user goes back to Web Browser application. Unfortunately, web browser
process was killed previously so the system has to kill another process (in our case it is Email application process, which is not used anymore) in order to locate Web Browser process and manage the stack memory. Now the user comes back to the Talk application and resumes his talk with his friend. Because of the saved states, going back procedure is fast and useful, because it remembers previous activities and its views.
This example shows, that it does not matter how many applications and processes are active or how much available memory is left, Android it manages fast and without a user interaction.
Fire and forget
For this method we just calls the child activity and flow the process. we are not getting any return value from the child activity. Here intent is just an event. It can have a target of an activity calss along with some date that's passed in via a Bundle.
refer : http://about-android.blogspot.com/2009/12/passing-data-or-parameter-to-another_02.html
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
3. Call the child Activity from mainactivity with the bundle
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
4. Get the Value from MainActivity in ChildActivity
this.getIntent().getExtras().getString("sample")
5. Toast the Message
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
}
}
Async callback, and correlationId
The calling-Activity has to provide a correlationId/request code to the Intent/event, before firing/raising it. This is then used by the sub-Activity to report it’s results back to the calling-Activity when it’s ready. The calling-Activity does not stop when it spawns the sub-Activity.
Please note that this sub-Activity is not "modal", that is, the calling Activity does not block, when startSubActivity() is called. So if you’re thinking that this is like a modal dialog box, where the calling-Activity will wait for the sub-Activity to produce a result, then you have to think of it differently.
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Call the child Activity from mainactivity with the bundle
Intent i = new Intent(this, ChildActivity.class);
startActivityForResult(i, 1);
4. Set the Value from ChildActivity To MainActivity
setResult(RESULT_OK, new Intent().putExtra("result", "value from Child Activity"));
finish();
5. Receive the value from ChildActiivity and Print the Message in onActivityResult Method
if (resultCode == RESULT_OK) {
String name = data.getStringExtra("result");
Toast.makeText(this, "You have chosen the City: " + " " + name,
Toast.LENGTH_LONG).show();
}
Source Code
MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.Toast;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Intent i = new Intent(this, ChildActivity.class);
startActivityForResult(i, 1);
}
protected void start(Intent intent) {
this.startActivityForResult(intent, 1);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (resultCode == RESULT_OK) {
String name = data.getStringExtra("result");
Toast.makeText(this, "You have chosen the Value: " + " " + name,
Toast.LENGTH_LONG).show();
}
super.onActivityResult(requestCode, resultCode, data);
}
}
ChildActivity.java
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, "Child Activity", Toast.LENGTH_LONG).show();
setResult(RESULT_OK, new Intent().putExtra("result", "value from Child Activity"));
finish();
}
}
Passing data or parameter to another Activity Android -2 ( Bundle Collection )
Passing data or parameter to another Activity Android - using Bundle
This following step helps to share the content using bundle.
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
3. Call the child Activity from mainactivity with the bundle
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
4. Get the Value from MainActivity in ChildActivity
this.getIntent().getExtras().getString("sample")
5. Toast the Message
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
}
}
This following step helps to share the content using bundle.
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
3. Call the child Activity from mainactivity with the bundle
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
4. Get the Value from MainActivity in ChildActivity
this.getIntent().getExtras().getString("sample")
5. Toast the Message
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Bundle bundle = new Bundle();
bundle.putString("sample", "this is the test commands");
bundle.putString("sample1", "this is the test commands1");
startActivity(new Intent(this, ChildActivity.class).putExtras(bundle));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, this.getIntent().getExtras().getString("sample"),
Toast.LENGTH_LONG).show();
Toast.makeText(this, this.getIntent().getExtras().getString("sample1"),
Toast.LENGTH_LONG).show();
}
}
Passing data from activity to activity using (Shared Preference ) in android
In this blog we have discussed shared preference and bundle collection. We can pass the value from parent activity to child activity using the bundled collection and shared preference.
1. Shared Preference
2. Bundle Collection
Shared Preference
Preferences are typically name value pairs. They can be stored as “Shared Preferences” across various activities in an application (note currently it cannot be shared across processes). First we need to put our value in to the context. And the context object lets you retrieve SharedPreferences through the method Context.getSharedPreferences().
1. Make a Shared Preference Collection
2. Retrieve Shared Preference Collection
Make a Shared Preference Collection
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
Retrieve Shared Preference Collection
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
prefsEditor.getString("sample", "DEFAULT VALUE");
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
3. Call the child Activity from mainactivity
startActivity(new Intent(this,ChildActivity.class));
4. Get the Value from MainActivity in ChildActivity
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
5. Toast the Message
Toast.makeText(this, myPrefs.getString("sample", "DEFAULT VALUE"), Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
startActivity(new Intent(this,ChildActivity.class));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
Toast.makeText(this, myPrefs.getString("sample", "DEFAULT VALUE"), Toast.LENGTH_LONG).show();
}
}
1. Shared Preference
2. Bundle Collection
Shared Preference
Preferences are typically name value pairs. They can be stored as “Shared Preferences” across various activities in an application (note currently it cannot be shared across processes). First we need to put our value in to the context. And the context object lets you retrieve SharedPreferences through the method Context.getSharedPreferences().
1. Make a Shared Preference Collection
2. Retrieve Shared Preference Collection
Make a Shared Preference Collection
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
Retrieve Shared Preference Collection
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
prefsEditor.getString("sample", "DEFAULT VALUE");
1. Create a two Activity [MainActivity & ChildActivity]
Activity : 1 - MainActivity public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
Activity : 2 - ChildActivity
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// TODO Put your code here
}
}
2. Define Your Shared collection SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
3. Call the child Activity from mainactivity
startActivity(new Intent(this,ChildActivity.class));
4. Get the Value from MainActivity in ChildActivity
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
5. Toast the Message
Toast.makeText(this, myPrefs.getString("sample", "DEFAULT VALUE"), Toast.LENGTH_LONG).show();
Full Source :MainActivity.java
import android.app.Activity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("sample", "this is test commands");
prefsEditor.commit();
startActivity(new Intent(this,ChildActivity.class));
}
}
-------ChildActivity.java----------
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
public class ChildActivity extends Activity {
/**
* @see android.app.Activity#onCreate(Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
SharedPreferences myPrefs = this.getSharedPreferences("contact", MODE_WORLD_READABLE);
Toast.makeText(this, myPrefs.getString("sample", "DEFAULT VALUE"), Toast.LENGTH_LONG).show();
}
}
Android Hello World Activity Sample
Android Hello World Activity Sample
1.Setup your Environment
2. Create an application
1. Setup Android Environment
a. Download latest Eclipse from http://eclipse.org/
b. update android Plug-in - Refer : http://about-android.blogspot.com/2009/11/about-android-first-of-all-android_09.html
c. Install Android SDK
Install new android SDK from the android site. Refer:http://about-android.blogspot.com/2009/11/about-android-first-of-all-android_09.html
D. Configure SDK with your Eclipse
Go to Window>Preference>Select Android > Browse "SDK PATH" > Apply
E. Create a Device / Emulator
Create emulator using the AVD manager
Open AVD Manager > New > Give Name/Target > Create AVD.
Android Environment has setted. Now we can create an android Application.
2. Create a " Hello World Apps "
a. Open Eclipse > File > Android Project.
b. Check the Android Folder Structure.
Go to Window > Show View > package Explorer
AndroidManifest.xml - It contains the overall application configuration.
src - Contains the java code like activites,service,broadcast receiver , etc,.
res - Contains the application resource
1. drawable - Icon,Image
2. raw - Sounds
3. menu - menu properties
4. values - application properites like title,color value, dropdown values.
5. layout - screen design
gen - Contains the R.java File which is used to map the resource and java src.
c. Run the application
Run > Run Android Application
D. Check the Emulator
Hope this is useful for create a hello world program.
Application Fundamentals
Application Fundamentals
Application Fundamentals
Android application archieve format is .apx
Each process has its own Java virtual machine (VM), so application code runs in isolation from the code of all other application
Android Application Components are Activites,Service,Broadcast receivers and Content providers
Activities
An application that has a visible UI is implemented with an activity. When a user selects an application from the home screen or application launcher, an activity is started.
Services
A service should be used for any application that needs to persist for a long time, such as a network monitor or update-checking application.
Content providers
You can think of content providers as a database server. A content provider's job is to manage access to persisted data, such as a SQLite database. If your application is very simple, you might not necessarily create a content provider. If you're building a larger application, or one that makes data available to multiple activities or applications, a content provider is the means of accessing your data.
Broadcast receivers
An Android application may be launched to process a element of data or respond to an event, such as the receipt of a text message.
AndroidManifest.xml - Configuration
An Android application, along with a file called AndroidManifest.xml, is deployed to a device.
AndroidManifest.xml contains the necessary configuration information to properly install it to the device. It includes the required class names and types of events the application is able to process, and the required permissions the application needs to run.
Project Folder Structure ;
1. src - It contain the java code
2. Resource - It contain the all resource with different floder
drawable - Icon
raw - Sounds
menu - Menu
values - Project Properties
layout - User interface Screens
3. gen - It contains the R.java file. You could not edit R.java manually. This have been generated automatically by understanding resource files etc.
4. AndroidManifest -It contains the Project properties
5. Android lib.
Architecture of Android
Architecture of Android :
1. Linux Kernal [ 2.6 Kernal - security, memory management, process management, network stack, and driver model]
2. Native Libraries [SQLite, WEBKit, OpenGL,..]
3. Runtime + Dalvik VM [.dex format, Lightweight VM, Efficient Dalvik Bytecode]
4. Application Framework [Activity Manager, Content Manager, Location Manager, ]
5. Application [ System Apps and Your Apps]
Linux Kernal
Android is based on the linux keral 2.6. From the Kernal 2.6 android using the hardward interaction layer
Android relies on Linux version 2.6 for core system services such as security, memory management, process management, network stack, and driver model. The kernel also acts as an abstraction layer between the hardware and the rest of the software stack.
Libraries
Android includes a set of C/C++ libraries used by various components of the Android system. These capabilities are exposed to developers through the Android application framework.
Android Runtime
Android includes a set of core libraries that provides most of the functionality available in the core libraries of the Java programming language.
Every Android application runs in its own process, with its own instance of the Dalvik virtual machine. Dalvik has been written so that a device can run multiple VMs efficiently. The Dalvik VM executes files in the Dalvik Executable (.dex) format which is optimized for minimal memory footprint. The VM is register-based, and runs classes compiled by a Java language compiler that have been transformed into the .dex format by the included "dx" tool.
The Dalvik VM relies on the Linux kernel for underlying functionality such as threading and low-level memory management.
Application Framework
Developers have full access to the same framework APIs used by the core applications. The application architecture is designed to simplify the reuse of components; any application can publish its capabilities and any other application may then make use of those capabilities (subject to security constraints enforced by the framework). This same mechanism allows components to be replaced by the user.
Underlying all applications is a set of services and systems, including:
* A rich and extensible set of Views that can be used to build an application, including lists, grids, text boxes, buttons, and even an embeddable web browser
* Content Providers that enable applications to access data from other applications (such as Contacts), or to share their own data
* A Resource Manager, providing access to non-code resources such as localized strings, graphics, and layout files
* A Notification Manager that enables all applications to display custom alerts in the status bar
* An Activity Manager that manages the lifecycle of applications and provides a common navigation backstack
Applications
Android will ship with a set of core applications including an email client, SMS program, calendar, maps, browser, contacts, and others. All applications are written using the Java programming language.
1. Linux Kernal [ 2.6 Kernal - security, memory management, process management, network stack, and driver model]
2. Native Libraries [SQLite, WEBKit, OpenGL,..]
3. Runtime + Dalvik VM [.dex format, Lightweight VM, Efficient Dalvik Bytecode]
4. Application Framework [Activity Manager, Content Manager, Location Manager, ]
5. Application [ System Apps and Your Apps]
Linux Kernal
Android is based on the linux keral 2.6. From the Kernal 2.6 android using the hardward interaction layer
Android relies on Linux version 2.6 for core system services such as security, memory management, process management, network stack, and driver model. The kernel also acts as an abstraction layer between the hardware and the rest of the software stack.
Libraries
Android includes a set of C/C++ libraries used by various components of the Android system. These capabilities are exposed to developers through the Android application framework.
Android Runtime
Android includes a set of core libraries that provides most of the functionality available in the core libraries of the Java programming language.
Every Android application runs in its own process, with its own instance of the Dalvik virtual machine. Dalvik has been written so that a device can run multiple VMs efficiently. The Dalvik VM executes files in the Dalvik Executable (.dex) format which is optimized for minimal memory footprint. The VM is register-based, and runs classes compiled by a Java language compiler that have been transformed into the .dex format by the included "dx" tool.
The Dalvik VM relies on the Linux kernel for underlying functionality such as threading and low-level memory management.
Application Framework
Developers have full access to the same framework APIs used by the core applications. The application architecture is designed to simplify the reuse of components; any application can publish its capabilities and any other application may then make use of those capabilities (subject to security constraints enforced by the framework). This same mechanism allows components to be replaced by the user.
Underlying all applications is a set of services and systems, including:
* A rich and extensible set of Views that can be used to build an application, including lists, grids, text boxes, buttons, and even an embeddable web browser
* Content Providers that enable applications to access data from other applications (such as Contacts), or to share their own data
* A Resource Manager, providing access to non-code resources such as localized strings, graphics, and layout files
* A Notification Manager that enables all applications to display custom alerts in the status bar
* An Activity Manager that manages the lifecycle of applications and provides a common navigation backstack
Applications
Android will ship with a set of core applications including an email client, SMS program, calendar, maps, browser, contacts, and others. All applications are written using the Java programming language.
About Android
About Android
First of all, Android operating system is running on the Linux kernel 2.6.27, meaning stronger security, improved stability and a range of core applications enhancements. Android provides packs SIM Application Toolkit 1.0 and features are auto-checking and repair of SD cardfile-system. Just like the iPhone OS 3.0, Android comes with the SDK that adds new APIs which help developers create better apps.
Some of the features are simply catchup of the iPhone’s, like a new virtual keyboard or improved mobile web browser. Others are designed to add more punch through flashier eye candy, like animated window transitions, smooth, accelerometer-based application rotations between portrait and landscape modes and overall polish of user interface elements.
Android Features:
* Application framework enabling reuse and replacement of components
* Dalvik virtual machine optimized for mobile devices. -
* Integrated browser based on the open source WebKit engine
* Optimized graphics powered by a custom 2D graphics library; 3D graphics based on the OpenGL ES 1.0 specification (hardware acceleration optional)
* SQLite for structured data storage
* Media support for common audio, video, and still image formats (MPEG4, H.264, MP3, AAC, AMR, JPG, PNG, GIF)
* GSM Telephony (hardware dependent)
* Bluetooth, EDGE, 3G, and WiFi (hardware dependent)
* Camera, GPS, compass, and accelerometer (hardware dependent)
* Rich development environment including a device emulator, tools for debugging, memory and performance profiling, and a plugin for the Eclipse IDE
* Support Other platform. Andriod NDK for c,c++ Developer and ASM for phython developers
Reference:
http://www.dalvikvm.com/ [1]
Top 10 features you’ll love about Android 1.5
- Smart virtual keyboard
- Home screen customizable with widgets
- Live Folders for quick-viewing your data
- Video recording and sharing
- Picasa image uploading
- Faster, standards-compliant browser
- Voice Search
- Stereo Bluetooth and hands-free calls
- Snappier overall performance
- Nice-to-haves
- Symbian OS from Symbian Ltd,
- RIM BlackBerry operating system
- iPhone OS from Apple Inc.
- Windows Mobile from Microsoft
- Linux operating system Palm webOS from Palm Inc.
How to Program Google Android in eclipse
You can bulid your android application using the powerful Eclipse environment. This part introduces Android application development with the Eclipse plug-in, otherwise known as Android Development Tools. This provides an introduction to Android development with a quick introduction to the platform, a tour of Android Development Tools, and includes the construction of "Hello World " example applications.
Prerequisites
This section provides to setup the right environment to develop the android application.
System Requirements
Eclipse Platform
Get the latest version of Eclipse 3.5 (Galileo) from http://www.eclipse.org/galileo/ (V3.5 was used in this tutorial).
Android Developer Tools
Get the latest version of Android SDK from http://developer.android.com/sdk/index.html (V2.0 was used in this tutorial).
Set The Android Environment
Steps to Install Eclipse and Add plugin
1. Get the Eclipse 3.5 archieve File from http://www.eclipse.org/galileo/
2. Extract the archieve File and run the Eclipse
3. Click Help->Install New software->Add
4. Type : Name :Android plug-in
Location: https://dl-ssl.google.com/android/eclipse/ (Refer:Image)
5. Install the Plug-in
6. Restart the Eclipse
Steps to Install Android SDK
1 : Download SDK from http://developer.android.com/sdk/index.html
2 : Run the Setup. - Goto the Commant pr
3 : You can see the popup (Android SDK and AVD Manager)
4 : If you encounter the following issue follow the solution, still if you have issue post your comments
----------------
Issue :Failed to fetch URL https://dl-ssl.google.com/andr oid/repository/repository.xml
Solution :
Step 1 : Run the Tool
Step 2 : Change settings options and checked in [ misc section "force https:// ... sources to be fetched using http://..." ]
Step 3 : Restart application
Step 4 : Ensure the Option Selected.
-----------------
5. Ensure your SDK is successfully updated.
Now the android environment is ready to create your application.
Develop Android Applications With Eclipse
You can develop your first android application using the below steps.
- Create Emulator using the android toolkit
- Configure Android SDK with your Eclipse
- Create a demo Application
1. Go to the SDK/tools/
2. Open a SDK Tool and run android.bat File
3. You can see the Android SDK and AVD Manager and Click "New"
4. Enter Device Name & Target Version and Click Create.
Configure Android SDK with your Eclipse
1. Open Eclipse
2. Go to preference : Window -> preference -> select Android
3. Browse and select the SDK Path (You can see the SDK version)
4. Apply and Close the preference popup
Create a demo Application
1. Open Eclipse and Create a new Android Project : File ->New -> Project -> Android Project
2. Enter the following data
1. project Name : Demo
2. Select Build Target : Android 1.1
3. Application Name : Demo
4. Package Name : com.demo
5. Create Activity : Demo
6. Min SDK Version : 2
3. Finally Click Finish
4. Check you application in project explorer
5. Run the your application : Run-> Run
About SDK Tool
You can find the following tools from your android sdk location
1. emulator – the emulator executable, that runs the APK files
2. adb – android debugger bridge, which is used to communicate with instances of the emulator
3. ddms – this is the debugger for the emulator executable
emulator – run your APK files
Run this program to launch the emulator that you can run your APK files in.
When you use plugins in your IDE to launch APK files, the emulator is started and the APK files are loaded it by the IDE.
otherwise you can using the below commands to install your application.
adb – install and uninstall APK files
The adb program is used to install APK files into the emulator that’s running.
Command : adb install
For example: “adb install SomeAndroidApp.apk”
To uninstall an APK file you have use adb to remove the file from the emulator itself.
For example: "adb shell rm data/app/SomeAndroidApp.apk"
*NOTE: To install or uninstall an APK file, please make sure that the emulator is already running.
ddms – debug and monitor your Android apps, and the emulator
This is the Android SDK emulator debugger. This is what you can do with your ebugger:
2. You can set the telephony status of your emulator. You can control the network data i/o speed and latency. You can even fake an incoming phone call into the emulator. You can even create an incoming SMS message.
3. You can take screenshots of the display of the emulator at any given time.
4. You can view the contents of the emulator’s filesystem. This is useful if you want to see where files are stored (that you download in your apps for example).
Here’s more information from Google Android docs on ddms.
The Eclipse plugin gives you full access to ddms functionality inside the IDE itself.
Emulator performance
To get an idea of what CPU speed your emulator is emulating try this:
1. start a shell session (adb shell),
2. then run "cat /proc/cpuinfo" to get the BogoMIPS.
Lanuch Application as Startup Application
In this article helps to lanuch your activity after the start your Phone.
1. Create a BroadcastReceiver Class and start your Main Activity
2. Set the User Permission for launch your application
3. Configure the Action and catgory in the intent-filter
4. Create a MainActivity
Create a BroadcastReceiver Class and start your Main Activity
This BroadcastReceiver is used to receive the action and start the your main activity
public class StartupActivity extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
}
}
Set the User Permission for launch your application
Config the " android.permission.RECEIVE_BOOT_COMPLETED " User Permission in the androidmanifest.xml
Configure the Action and catgory in the intent-filter
Set the following values for android and category
Action : android.intent.action.BOOT_COMPLETED
Category : android.intent.category.DEFAULT
Create a MainActivity
Create a MainActivity and write your logic there
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
}
}
Sample Code
MainActivity.java
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, "Welcome to Application", Toast.LENGTH_LONG)
.show();
finish();
}
}
BroadcastReceiver.java
public class StartupActivity extends BroadcastReceiver {
/**
* @see android.content.BroadcastReceiver#onReceive(Context,Intent)
*/
@Override
public void onReceive(Context context, Intent intent) {
Intent i = new Intent(context, MainActivity.class);
i.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(i);
}
}
Androidmanifest.xml
<?xml version="1.0" encoding="UTF-8"?>
<manifest android:versionCode="1" android:versionName="1.0"
package="com.lanuchactivity" xmlns:android="http://schemas.android.com/apk/res/android">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:label="@string/app_name" android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name=".StartupActivity">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED"></action>
<category android:name="android.intent.category.DEFAULT"></category>
</intent-filter>
</receiver>
</application>
<uses-sdk android:minSdkVersion="7" />
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED"></uses-permission>
</manifest>
1. Create a BroadcastReceiver Class and start your Main Activity
2. Set the User Permission for launch your application
3. Configure the Action and catgory in the intent-filter
4. Create a MainActivity
Create a BroadcastReceiver Class and start your Main Activity
This BroadcastReceiver is used to receive the action and start the your main activity
public class StartupActivity extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
}
}
Set the User Permission for launch your application
Config the " android.permission.RECEIVE_BOOT_COMPLETED " User Permission in the androidmanifest.xml
Configure the Action and catgory in the intent-filter
Set the following values for android and category
Action : android.intent.action.BOOT_COMPLETED
Category : android.intent.category.DEFAULT
Create a MainActivity
Create a MainActivity and write your logic there
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
}
}
Sample Code
MainActivity.java
public class MainActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Toast.makeText(this, "Welcome to Application", Toast.LENGTH_LONG)
.show();
finish();
}
}
BroadcastReceiver.java
public class StartupActivity extends BroadcastReceiver {
/**
* @see android.content.BroadcastReceiver#onReceive(Context,Intent)
*/
@Override
public void onReceive(Context context, Intent intent) {
Intent i = new Intent(context, MainActivity.class);
i.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(i);
}
}
Androidmanifest.xml
<?xml version="1.0" encoding="UTF-8"?>
<manifest android:versionCode="1" android:versionName="1.0"
package="com.lanuchactivity" xmlns:android="http://schemas.android.com/apk/res/android">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:label="@string/app_name" android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name=".StartupActivity">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED"></action>
<category android:name="android.intent.category.DEFAULT"></category>
</intent-filter>
</receiver>
</application>
<uses-sdk android:minSdkVersion="7" />
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED"></uses-permission>
</manifest>
Saturday, August 6, 2011
android list view with adepter , aleart dialoge box EXAMPLE IN ANDROID
/** Called when the activity is first created. */
TextView selection;
private ListView lv1;
private String lv_arr[]={"Android","iPhone","
"Brazil", "Cote d'Ivoire", "Cameroon",
"Chile", "Costa Rica", "Denmark",
"England", "France", "Germany",
"Ghana", "Greece", "Honduras",
"Italy", "Japan", "Netherlands",
"New Zealand", "Nigeria", "North Korea",
"Paraguay", "Portugal","Serbia",
"Slovakia", "Slovenia", "South Africa",
"South Korea", "Spain", "Switzerland",
"United States", "Uruguay"};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(
setContentView(R.layout.main);
selection=(TextView)
lv1=(ListView)findViewById(R.
// By using setAdpater method in listview we an add string array in list.
lv1.setAdapter(new ArrayAdapter
lv1.setTextFilterEnabled(true)
lv1.setOnItemClickListener(new OnItemClickListener()
{
@Override
public void onItemClick(AdapterView a, View v, int position, long id) {
AlertDialog.Builder adb=new AlertDialog.Builder(
adb.setTitle("
adb.setMessage("Selected Item is = "+lv1.getItemAtPosition(
adb.setPositiveButton("Ok", null);
adb.show();
}
});
}
}
............
main.xml
...............
--
krishnachary
MAPS AND LOCATIONS EXAMPLE
1 ONLY ON LOCATION EXAMPLE , 2 MAPS AND LOCATIONS EXAMPLE
==============================
1 ONLY ON LOCATION EXAMPLE
------------------------------ ------------------
package com.gps;
import android.app.Activity;
import android.content.Context;
import android.location.Location;
import android.location. LocationListener;
import android.location. LocationManager;
import android.os.Bundle;
import android.view.View;
import android.view.View. OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class GPSLocation extends Activity {
protected LocationManager locationManager;
protected Button retrieveLocationButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate( savedInstanceState);
setContentView(R.layout.main);
retrieveLocationButton = (Button) findViewById(R.id.btn);
locationManager = (LocationManager) getSystemService(Context. LOCATION_SERVICE);
locationManager. requestLocationUpdates( LocationManager.GPS_PROVIDER, 0,0,new MyLocationListener());
retrieveLocationButton. setOnClickListener(new OnClickListener() {
public void onClick(View v) {
showCurrentLocation();
}
});
}
protected void showCurrentLocation() {
Location location = locationManager. getLastKnownLocation( LocationManager.GPS_PROVIDER);
if (location != null) {
String message = String.format(
"Current Location \n Longitude: %s \n Latitude: %s",location.getLongitude(), location.getLatitude()
);
Toast.makeText(GPSLocation. this, message,Toast.LENGTH_LONG). show();
}
}
private class MyLocationListener implements LocationListener {
public void onLocationChanged(Location location) {
String message = String.format( "New Location \n Longitude: %s \n Latitude: %s",location.getLongitude(), location.getLatitude());
Toast.makeText(GPSLocation. this, message, Toast.LENGTH_LONG).show();
}
public void onStatusChanged(String s, int i, Bundle b) {
Toast.makeText(GPSLocation. this, "Provider status changed",Toast.LENGTH_LONG). show();
}
public void onProviderDisabled(String s) {
Toast.makeText(GPSLocation. this,
"Provider disabled by the user. GPS turned off",Toast.LENGTH_LONG).show() ;
}
public void onProviderEnabled(String s) {
Toast.makeText(GPSLocation. this,
"Provider enabled by the user. GPS turned on",Toast.LENGTH_LONG).show();
}
}
}
***************************
2 MAPS AND LOCATIONS EXAMPLE
------------------------------ --------------------
mapsimple example
----------------------------
package com.map;
import com.google.android.maps. MapActivity;
import com.google.android.maps. MapView;
import android.os.Bundle;
public class HelloGoogleMaps extends MapActivity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate( savedInstanceState);
setContentView(R.layout.main);
MapView mapView = (MapView) findViewById(R.id.mapview);
mapView. setBuiltInZoomControls(true);
}
/*DefaultHttpClient client = new DefaultHttpClient();
String proxyHost = android.net.Proxy.getHost( this);
if (proxyHost !=null) {
int proxyPort = android.net.Proxy.getPort( this);
HttpHost proxy = new HttpHost("*********", ***);
client.getParams(). setParameter(ConnRoutePNames. DEFAULT_PROXY, proxy);
}
}
*/
@Override
protected boolean isRouteDisplayed() {
return false;
}
}
*****************************
mapexample 2
-----------------------
package com.wissen.android;
import java.util.List;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics. BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Point;
import android.graphics.drawable. Drawable;
import android.location.Location;
import android.location. LocationListener;
import android.location. LocationManager;
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.ViewGroup;
import android.view.ViewGroup. LayoutParams;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ZoomControls;
import com.google.android.maps. GeoPoint;
import com.google.android.maps. MapActivity;
import com.google.android.maps. MapController;
import com.google.android.maps. MapView;
import com.google.android.maps. Overlay;
public class Hello extends MapActivity implements LocationListener {
/** Called when the activity is first created. */
EditText txted = null;
Button btnSimple = null;
MapView gMapView = null;
MapController mc = null;
Drawable defaultMarker = null;
GeoPoint gp = null;
double latitude = 17.518344, longitude = 78.442383;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate( savedInstanceState);
setContentView(R.layout.main);
// Creating TextBox displying Lat, Long
txted = (EditText) findViewById(R.id.id1);
String currentLocation = "Lat: " + latitude + " Lng: " + longitude;
txted.setText(currentLocation) ;
// Creating and initializing Map
gMapView = (MapView) findViewById(R.id.myGMap);
gp = new GeoPoint((int) (latitude * 1000000), (int) (longitude * 1000000));
//gMapView.setSatellite(true);
mc = gMapView.getController();
mc.setCenter(gp);
mc.setZoom(14);
// Add a location mark
MyLocationOverlay myLocationOverlay = new MyLocationOverlay();
List list = gMapView.getOverlays();
list.add(myLocationOverlay);
// Adding zoom controls to Map
ZoomControls zoomControls = (ZoomControls) gMapView.getZoomControls();
zoomControls.setLayoutParams( new ViewGroup.LayoutParams( LayoutParams.WRAP_CONTENT,
LayoutParams.WRAP_CONTENT));
gMapView.addView(zoomControls) ;
gMapView.displayZoomControls( true);
// Getting locationManager and reflecting changes over map if distance travel by
// user is greater than 500m from current location.
LocationManager lm = (LocationManager) getSystemService(Context. LOCATION_SERVICE);
lm.requestLocationUpdates( LocationManager.GPS_PROVIDER, 1000L, 500.0f, this);
}
/* This method is called when use position will get changed */
public void onLocationChanged(Location location) {
if (location != null) {
double lat = location.getLatitude();
double lng = location.getLongitude();
String currentLocation = "Lat: " + lat + " Lng: " + lng;
txted.setText(currentLocation) ;
gp = new GeoPoint((int) lat * 1000000, (int) lng * 1000000);
mc.animateTo(gp);
}
}
public void onProviderDisabled(String provider) {
// required for interface, not used
}
public void onProviderEnabled(String provider) {
// required for interface, not used
}
public void onStatusChanged(String provider, int status, Bundle extras) {
// required for interface, not used
}
protected boolean isRouteDisplayed() {
// TODO Auto-generated method stub
return false;
}
/* User can zoom in/out using keys provided on keypad */
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_I) {
gMapView.getController(). setZoom(gMapView.getZoomLevel( ) + 1);
return true;
} else if (keyCode == KeyEvent.KEYCODE_O) {
gMapView.getController(). setZoom(gMapView.getZoomLevel( ) - 1);
return true;
} else if (keyCode == KeyEvent.KEYCODE_S) {
gMapView.setSatellite(true);
return true;
} else if (keyCode == KeyEvent.KEYCODE_T) {
gMapView.setTraffic(true);
return true;
}
return false;
}
/* Class overload draw method which actually plot a marker,text etc. on Map */
protected class MyLocationOverlay extends com.google.android.maps. Overlay {
@Override
public boolean draw(Canvas canvas, MapView mapView, boolean shadow, long when) {
Paint paint = new Paint();
shadow=true;
super.draw(canvas, mapView, shadow);
// Converts lat/lng-Point to OUR coordinates on the screen.
Point p = new Point();
mapView.getProjection(). toPixels(gp, p);
paint.setStrokeWidth(1);
paint.setARGB(255, 255, 255, 255);
paint.setStyle(Paint.Style. STROKE);
Bitmap bmp = BitmapFactory.decodeResource( getResources(), R.drawable.marker);
canvas.drawBitmap(bmp, p.x,p.y, paint);
canvas.drawText("I am here...", p.x, p.y, paint);
return true;
}
}
}
****************************** *********************
mapexample direction of locations
------------------------------ ------------------
package com.directions;
import java.util.ArrayList;
import java.util.List;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Point;
import android.graphics.drawable. Drawable;
import android.os.Bundle;
import android.view.KeyEvent;
import android.widget.Toast;
import com.google.android.maps. GeoPoint;
import com.google.android.maps. ItemizedOverlay;
import com.google.android.maps. MapActivity;
import com.google.android.maps. MapView;
import com.google.android.maps. MyLocationOverlay;
import com.google.android.maps. OverlayItem;
import com.google.android.maps. Projection;
public class Directions extends MapActivity {
private MapView map=null;
private MyLocationOverlay me=null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate( savedInstanceState);
setContentView(R.layout.main);
map=(MapView)findViewById(R. id.map);
map.getController().setCenter( getPoint(17.390777,78.4762));/ /Center point as Abdies
map.getController().setZoom( 14);
map.setBuiltInZoomControls( true);
Drawable marker=getResources(). getDrawable(R.drawable.marker) ;
marker.setBounds(0, 0, marker.getIntrinsicWidth(), marker.getIntrinsicHeight());
map.getOverlays().add(new SitesOverlay(marker));
me=new MyLocationOverlay(this, map);
map.getOverlays().add(me);
}
@Override
public void onResume() {
super.onResume();
me.enableCompass();
}
@Override
public void onPause() {
super.onPause();
me.disableCompass();
}
@Override
protected boolean isRouteDisplayed() {
return(false);
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_S) {
map.setSatellite(!map. isSatellite());
return(true);
}
else if (keyCode == KeyEvent.KEYCODE_Z) {
map.displayZoomControls(true);
return(true);
}
return(super.onKeyDown( keyCode, event));
}
private GeoPoint getPoint(double lat, double lon) {
return(new GeoPoint((int)(lat*1000000.0),
(int)(lon*1000000.0)));
}
private class SitesOverlay extends ItemizedOverlay {
private List items=new ArrayList();
private Drawable marker=null;
private GeoPoint abdis=getPoint(17.390777,78. 4762); // starting point Abdis
private GeoPoint lakdi=getPoint(17.398804,78. 462639); // lakdikapool
private GeoPoint panga=getPoint(17.425994,78. 451481);// panjagutta
private GeoPoint ameer=getPoint(17.439424,78. 444958);// Ameerpet
public SitesOverlay(Drawable marker) {
super(marker);
this.marker=marker;
items.add(new OverlayItem(getPoint(17. 390777,78.4762),"HYD", "Abdis"));
items.add(new OverlayItem(getPoint(17. 398804,78.462639),"HYD"," Lakdikapool"));
items.add(new OverlayItem(getPoint(17. 425994,78.451481),"HYD"," Panjagutta"));
items.add(new OverlayItem(getPoint(17. 439424,78.444958),"HYD"," Ameerpet"));
populate();
}
@Override
protected OverlayItem createItem(int i) {
return(items.get(i));
}
@Override
public void draw(Canvas canvas, MapView mapView,
boolean shadow) {
super.draw(canvas, mapView, shadow);
Projection projection = mapView.getProjection();
Paint paint = new Paint();
paint.setColor(000);
paint.setStrokeWidth(5);
paint.setAlpha(120);
Point point = new Point();
Point point2 = new Point();
Point point3 = new Point();
Point point4 = new Point();
Point point5 = new Point();
projection.toPixels(abdis, point);
projection.toPixels(lakdi, point2);
projection.toPixels(panga, point3);
projection.toPixels(ameer, point4);
canvas.drawLine(point.x, point.y, point2.x, point2.y, paint);// from Abdis to Lakdikapool
canvas.drawLine(point2.x, point2.y, point3.x, point3.y, paint);// from Lakdikapool to panjagutta
canvas.drawLine(point3.x, point3.y, point4.x, point4.y, paint);// from panjagutta to Ameerpet
super.draw(canvas, mapView, shadow);
boundCenterBottom(marker);
}
@Override
protected boolean onTap(int i) {
Toast.makeText(Directions. this,items.get(i).getSnippet() ,Toast.LENGTH_SHORT).show();
return(true);
}
@Override
public int size() {
return(items.size());
}
}
}
--
krishnachary
==============================
1 ONLY ON LOCATION EXAMPLE
------------------------------
package com.gps;
import android.app.Activity;
import android.content.Context;
import android.location.Location;
import android.location.
import android.location.
import android.os.Bundle;
import android.view.View;
import android.view.View.
import android.widget.Button;
import android.widget.Toast;
public class GPSLocation extends Activity {
protected LocationManager locationManager;
protected Button retrieveLocationButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(
setContentView(R.layout.main);
retrieveLocationButton = (Button) findViewById(R.id.btn);
locationManager = (LocationManager) getSystemService(Context.
locationManager.
retrieveLocationButton.
public void onClick(View v) {
showCurrentLocation();
}
});
}
protected void showCurrentLocation() {
Location location = locationManager.
if (location != null) {
String message = String.format(
"Current Location \n Longitude: %s \n Latitude: %s",location.getLongitude(), location.getLatitude()
);
Toast.makeText(GPSLocation.
}
}
private class MyLocationListener implements LocationListener {
public void onLocationChanged(Location location) {
String message = String.format( "New Location \n Longitude: %s \n Latitude: %s",location.getLongitude(), location.getLatitude());
Toast.makeText(GPSLocation.
}
public void onStatusChanged(String s, int i, Bundle b) {
Toast.makeText(GPSLocation.
}
public void onProviderDisabled(String s) {
Toast.makeText(GPSLocation.
"Provider disabled by the user. GPS turned off",Toast.LENGTH_LONG).show()
}
public void onProviderEnabled(String s) {
Toast.makeText(GPSLocation.
"Provider enabled by the user. GPS turned on",Toast.LENGTH_LONG).show();
}
}
}
***************************
2 MAPS AND LOCATIONS EXAMPLE
------------------------------
mapsimple example
----------------------------
package com.map;
import com.google.android.maps.
import com.google.android.maps.
import android.os.Bundle;
public class HelloGoogleMaps extends MapActivity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(
setContentView(R.layout.main);
MapView mapView = (MapView) findViewById(R.id.mapview);
mapView.
}
/*DefaultHttpClient client = new DefaultHttpClient();
String proxyHost = android.net.Proxy.getHost(
if (proxyHost !=null) {
int proxyPort = android.net.Proxy.getPort(
HttpHost proxy = new HttpHost("*********", ***);
client.getParams().
}
}
*/
@Override
protected boolean isRouteDisplayed() {
return false;
}
}
*****************************
mapexample 2
-----------------------
package com.wissen.android;
import java.util.List;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Point;
import android.graphics.drawable.
import android.location.Location;
import android.location.
import android.location.
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.ViewGroup;
import android.view.ViewGroup.
import android.widget.Button;
import android.widget.EditText;
import android.widget.ZoomControls;
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
public class Hello extends MapActivity implements LocationListener {
/** Called when the activity is first created. */
EditText txted = null;
Button btnSimple = null;
MapView gMapView = null;
MapController mc = null;
Drawable defaultMarker = null;
GeoPoint gp = null;
double latitude = 17.518344, longitude = 78.442383;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(
setContentView(R.layout.main);
// Creating TextBox displying Lat, Long
txted = (EditText) findViewById(R.id.id1);
String currentLocation = "Lat: " + latitude + " Lng: " + longitude;
txted.setText(currentLocation)
// Creating and initializing Map
gMapView = (MapView) findViewById(R.id.myGMap);
gp = new GeoPoint((int) (latitude * 1000000), (int) (longitude * 1000000));
//gMapView.setSatellite(true);
mc = gMapView.getController();
mc.setCenter(gp);
mc.setZoom(14);
// Add a location mark
MyLocationOverlay myLocationOverlay = new MyLocationOverlay();
List
list.add(myLocationOverlay);
// Adding zoom controls to Map
ZoomControls zoomControls = (ZoomControls) gMapView.getZoomControls();
zoomControls.setLayoutParams(
LayoutParams.WRAP_CONTENT));
gMapView.addView(zoomControls)
gMapView.displayZoomControls(
// Getting locationManager and reflecting changes over map if distance travel by
// user is greater than 500m from current location.
LocationManager lm = (LocationManager) getSystemService(Context.
lm.requestLocationUpdates(
}
/* This method is called when use position will get changed */
public void onLocationChanged(Location location) {
if (location != null) {
double lat = location.getLatitude();
double lng = location.getLongitude();
String currentLocation = "Lat: " + lat + " Lng: " + lng;
txted.setText(currentLocation)
gp = new GeoPoint((int) lat * 1000000, (int) lng * 1000000);
mc.animateTo(gp);
}
}
public void onProviderDisabled(String provider) {
// required for interface, not used
}
public void onProviderEnabled(String provider) {
// required for interface, not used
}
public void onStatusChanged(String provider, int status, Bundle extras) {
// required for interface, not used
}
protected boolean isRouteDisplayed() {
// TODO Auto-generated method stub
return false;
}
/* User can zoom in/out using keys provided on keypad */
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_I) {
gMapView.getController().
return true;
} else if (keyCode == KeyEvent.KEYCODE_O) {
gMapView.getController().
return true;
} else if (keyCode == KeyEvent.KEYCODE_S) {
gMapView.setSatellite(true);
return true;
} else if (keyCode == KeyEvent.KEYCODE_T) {
gMapView.setTraffic(true);
return true;
}
return false;
}
/* Class overload draw method which actually plot a marker,text etc. on Map */
protected class MyLocationOverlay extends com.google.android.maps.
@Override
public boolean draw(Canvas canvas, MapView mapView, boolean shadow, long when) {
Paint paint = new Paint();
shadow=true;
super.draw(canvas, mapView, shadow);
// Converts lat/lng-Point to OUR coordinates on the screen.
Point p = new Point();
mapView.getProjection().
paint.setStrokeWidth(1);
paint.setARGB(255, 255, 255, 255);
paint.setStyle(Paint.Style.
Bitmap bmp = BitmapFactory.decodeResource(
canvas.drawBitmap(bmp, p.x,p.y, paint);
canvas.drawText("I am here...", p.x, p.y, paint);
return true;
}
}
}
******************************
mapexample direction of locations
------------------------------
package com.directions;
import java.util.ArrayList;
import java.util.List;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Point;
import android.graphics.drawable.
import android.os.Bundle;
import android.view.KeyEvent;
import android.widget.Toast;
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
import com.google.android.maps.
public class Directions extends MapActivity {
private MapView map=null;
private MyLocationOverlay me=null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(
setContentView(R.layout.main);
map=(MapView)findViewById(R.
map.getController().setCenter(
map.getController().setZoom(
map.setBuiltInZoomControls(
Drawable marker=getResources().
marker.setBounds(0, 0, marker.getIntrinsicWidth(),
map.getOverlays().add(new SitesOverlay(marker));
me=new MyLocationOverlay(this, map);
map.getOverlays().add(me);
}
@Override
public void onResume() {
super.onResume();
me.enableCompass();
}
@Override
public void onPause() {
super.onPause();
me.disableCompass();
}
@Override
protected boolean isRouteDisplayed() {
return(false);
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_S) {
map.setSatellite(!map.
return(true);
}
else if (keyCode == KeyEvent.KEYCODE_Z) {
map.displayZoomControls(true);
return(true);
}
return(super.onKeyDown(
}
private GeoPoint getPoint(double lat, double lon) {
return(new GeoPoint((int)(lat*1000000.0),
(int)(lon*1000000.0)));
}
private class SitesOverlay extends ItemizedOverlay
private List
private Drawable marker=null;
private GeoPoint abdis=getPoint(17.390777,78.
private GeoPoint lakdi=getPoint(17.398804,78.
private GeoPoint panga=getPoint(17.425994,78.
private GeoPoint ameer=getPoint(17.439424,78.
public SitesOverlay(Drawable marker) {
super(marker);
this.marker=marker;
items.add(new OverlayItem(getPoint(17.
items.add(new OverlayItem(getPoint(17.
items.add(new OverlayItem(getPoint(17.
items.add(new OverlayItem(getPoint(17.
populate();
}
@Override
protected OverlayItem createItem(int i) {
return(items.get(i));
}
@Override
public void draw(Canvas canvas, MapView mapView,
boolean shadow) {
super.draw(canvas, mapView, shadow);
Projection projection = mapView.getProjection();
Paint paint = new Paint();
paint.setColor(000);
paint.setStrokeWidth(5);
paint.setAlpha(120);
Point point = new Point();
Point point2 = new Point();
Point point3 = new Point();
Point point4 = new Point();
Point point5 = new Point();
projection.toPixels(abdis, point);
projection.toPixels(lakdi, point2);
projection.toPixels(panga, point3);
projection.toPixels(ameer, point4);
canvas.drawLine(point.x, point.y, point2.x, point2.y, paint);// from Abdis to Lakdikapool
canvas.drawLine(point2.x, point2.y, point3.x, point3.y, paint);// from Lakdikapool to panjagutta
canvas.drawLine(point3.x, point3.y, point4.x, point4.y, paint);// from panjagutta to Ameerpet
super.draw(canvas, mapView, shadow);
boundCenterBottom(marker);
}
@Override
protected boolean onTap(int i) {
Toast.makeText(Directions.
return(true);
}
@Override
public int size() {
return(items.size());
}
}
}
--
krishnachary
Subscribe to:
Posts (Atom)