There is a post in The official Google Code blog, discuss about Getting started on the Google+ API. It's a exercise to query and parse Google+ JSON using Google+ API.
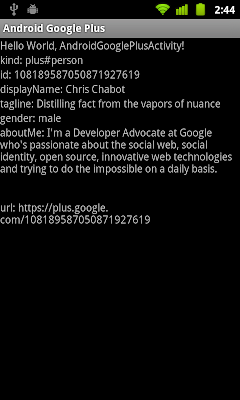
To use Google+ API, you have to
note: have to modify AndroidManifest.xml to grant permission of "android.permission.INTERNET".
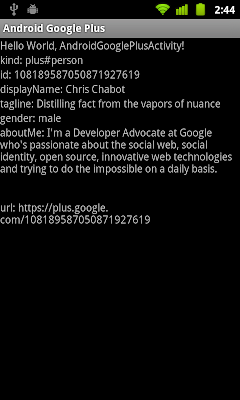
To use Google+ API, you have to
package com.exercise.AndroidGooglePlus;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.Bundle;
import android.text.Html;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidGooglePlusActivity extends Activity {
TextView item_kind;
TextView item_id;
TextView item_displayName;
TextView item_tagline;
TextView item_gender;
TextView item_aboutMe;
TextView item_url;
final static String GooglePlus_url
= "https://www.googleapis.com/plus/v1/people/108189587050871927619?key=<your own Google+ API key>";
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
item_kind = (TextView)findViewById(R.id.item_kind);
item_id = (TextView)findViewById(R.id.item_id);
item_displayName = (TextView)findViewById(R.id.item_displayName);
item_tagline = (TextView)findViewById(R.id.item_tagline);
item_gender = (TextView)findViewById(R.id.item_gender);
item_aboutMe = (TextView)findViewById(R.id.item_aboutMe);
item_url = (TextView)findViewById(R.id.item_url);
String googlePlusResult = QueryGooglePlus();
ParseGooglePlusJSON(googlePlusResult);
}
String QueryGooglePlus(){
String qResult = null;
HttpClient httpClient = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(GooglePlus_url);
try {
HttpEntity httpEntity = httpClient.execute(httpGet).getEntity();
if (httpEntity != null){
InputStream inputStream = httpEntity.getContent();
Reader in = new InputStreamReader(inputStream);
BufferedReader bufferedreader = new BufferedReader(in);
StringBuilder stringBuilder = new StringBuilder();
String stringReadLine = null;
while ((stringReadLine = bufferedreader.readLine()) != null) {
stringBuilder.append(stringReadLine + "\n");
}
qResult = stringBuilder.toString();
}
} catch (ClientProtocolException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
} catch (IOException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
}
return qResult;
}
void ParseGooglePlusJSON(String json){
if(json != null){
try {
JSONObject JsonObject = new JSONObject(json);
item_kind.setText("kind: " + JsonObject.getString("kind"));
item_id.setText("id: " + JsonObject.getString("id"));
item_displayName.setText("displayName: " + JsonObject.getString("displayName"));
item_tagline.setText("tagline: " + JsonObject.getString("tagline"));
item_gender.setText("gender: " + JsonObject.getString("gender"));
item_aboutMe.setText("aboutMe: " + Html.fromHtml(JsonObject.getString("aboutMe")));
item_url.setText("url: " + JsonObject.getString("url"));
Toast.makeText(AndroidGooglePlusActivity.this,
"finished",
Toast.LENGTH_LONG).show();
} catch (JSONException e) {
e.printStackTrace();
Toast.makeText(AndroidGooglePlusActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
}
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:id="@+id/item_kind"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_id"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_displayName"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_tagline"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_gender"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_aboutMe"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/item_url"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
note: have to modify AndroidManifest.xml to grant permission of "android.permission.INTERNET".
No comments:
Post a Comment